This is simply a collection of online resources related to JavaScript applications development.
This collection of JS appdev resources is a bit of a grab bag, some resources for noobs, some more advanced, and there are some links to useful tools here as well (e.g. to test screen capabilities, to test media query support, etc.)
JavaScript language
- David Flanagan's book "JavaScript:The Definitive Guide" is incredibly comprehensive, an essential item for your JavaScript library
- Another good book (this one online and free) is "Eloquent JavaScript"
- MDN has a useful JavaScript intro and reference
- For a JS refresher see MDN's A re-introduction to JavaScript or typedarray.org's JavaScript refresh
- Microsoft's Create Advanced Web Applications With Object-Oriented Techniques
- Thomas Hunter has a nice presentation on The JavaScript event loop
- Video: Misko Hevery's "Introduction to JavaScript and Browser DOM"
- Video: 2 versions of Paul Irish's presentation on js appdev "tooling ecosystem": here and here
- tutsplus.com post on the essentials of writing high quality javascript
- jQuery has a fairly in-depth JavaScript style guide
- I'll include Douglas Crockford's JS videos, ok for a JS intro, though I personally find his prescriptions too dogmatic
General JavaScript dev ("ecosystem")
- Medium's "State of the Art JavaScript in 2016"
- Telerik's post "What To Expect From JavaScript In 2016 – Frameworks" lowballs React (IMHO) but is still interesting
- Rebecca Murphey has a 2015 update to her useful post on essential JS skills for front-end developers
- dailyjs.com's annual survey of web developers is always interesting, here's 2014 and 2013 (why no 2015 post?).
- trends.builtwith.com's JavaScript usage statistics
- for data on browser market share you can try Netmarketshare, Statcounter or w3Counter
Standards and browser support
- Check for browser support for a feature at caniuse.com
- Here's a mobile HTML5 compatibility table that tells you which standards each major mobile browser actually supports
- See if a feature is ready to use or if you should steer clear of it, find good polyfills, get links to good resources on a feature, and more at html5please.com
- Read the standards in all their glory at w3.org/TR
- here is an annotated ECMAScript 5.1 specification
- Kangax maintains a compatibility table for different ECMAScript versions
Architecture
- Addy Osmani's slide deck for his talk "Tools for jQuery Application Architecture"
- Osmani also has an excellent speakerdeck on decoupling JavaScript
- MSDN has a book-length analysis of its Project Silk SPA design/architecture
- Osmani's "Learning JavaScript Design Patterns" book available for purchase from O'Reilly or online
- Stefanov also has a good JS design patterns book "JavaScript Patterns"
- tutsplus.com tutorial on dependency injection
- Addy Osmani's post explaining MVVM for JavaScript developers
- While this Safari books online article "Decoupling Backbone applications with pubsub" focuses on Backbone implementation the pub/sub pattern is universal and often useful for keeping your applications loosely coupled
Front-end development
- The awesome list for front-end development is a great starting point and has way more useful resources than this list
- Fernando Sousa's comprehensive collection of resources for frontend development is a bit older but still useful
- and Smashing's "List of talks to help you become a better front end engineer in 2013" is also somewhat dated but still very useful
- Tait Brown's front-end development guidelines helps you do things properly
- And these 3 posts help you do things efficiently:
- Smashing has another oldy but goody covering an essential skill: The principles of cross browser CSS coding
- This comparison of Angular/Ember/React will give noobs some current (2016) background on front-end frameworks and libs (I'll probably soon create a primer on React followed by something on React Native, great stuff...)
jQuery
- Rebecca Murphey's JQuery Fundamentals (original)
- Smashing's article on essentialjQuery plugin patterns
- Miller H. Borges Medeiros has an interesting post http://blog.millermedeiros.com/stop-writing-plugins-start-writing-components/
- http://dumitruglavan.com/jquery-performance-tips-cheat-sheet/
Performance
- Yahoo's rules for optimal performance is a good overview of issues and fixes.
- Google's Page Speed Insights analyzes your page's speed on desktop and mobile, suggests enhancements, and has guides to eliminating bottlenecks.
- Another web page analyzer is webpagetest.org
- Google Developers video "Getting Your PageSpeed Score Up" covers the basics of optimization (minification, concatenation, etc.) and "Supercharging page load (100 Days of Google Dev)" is similar but a bit more advanced.
- Google dev Ilya Grigorik has a great post on "Analyzing critical rendering path performance". For a deeper dive that especially focuses on latency with mobile see his video from the 2013 Velocity conference "Optimizing the Critical Rendering Path for Instant Mobile Websites"".
- Smashing has a post on writing fast memory efficient JavaScript
- html5rocks.com post on improving the performance of your HTML5 app
- MDN article on JavaScript memory management
- IBM developerworks paper on understanding JavaScript memory leaks
- http://gent.ilcore.com/2011/03/how-not-to-trigger-layout-in-webkit.html
- tutsplus.com post on the pros and cons of nesting functions
- http://dumitruglavan.com/jquery-performance-tips-cheat-sheet/
- John McCutchan's "Use forensics and detective work to solve JavaScript performance mysteries"
- Smashing's "How To Make Your Websites Faster On Mobile Devices"
Mobile
- HTML5rocks entry point for mobile web
- For mobile you must understand the viewport and quirksmode's Tale of two viewports is very useful, as are the tables in its "Browser Compatibility -- viewports" post
- David Calhoun's Using Mobile-specific HTML, CSS, and JavaScript is a little old but has still-useful info
- Maximiliano Firtman is an excellent source on the state of mobile browsers and mobile application development, here are a couple of video examples: "Android and the eternal dying mobile browser", and "The mobile web challenges"
- These next few bullets relate to performance on mobile. This first is a bit old now but still interesting: sealedabstract.com post "Why mobile web apps are slow"
- here's a repeat of Smashing's "How To Make Your Websites Faster On Mobile Devices"
- This readwrite.com December 2014 article "What Google Has In Store For The Mobile Web" can give hope to web developers concerned about mobile performance
- This New York Times mobile-focused tech predictions for 2013 is a bit dated but still interesting
- Smashing has a nice intro to responsive web design
- and here are some sites with info on mobile screen dimensions such as px height and width, physical px v. CSS px, pixel ratio, etc.: http://mydevice.io/devices/, http://viewportsizes.com/, http://www.canbike.org/CSSpixels/
Some screen check tools
Tools for querying screen capabilities:
- quirksmode's width test gives screen info and is especially useful for mobile because it lets you tweak the viewport setting
- more screen info including dpi is at http://dpi.lv/
- check media query support
HTML and CSS
You can't create web applications without a solid knowledge of both HTML and CSS, so here are some related links:
- Mozilla has a very nice intro to the DOM, which itself has useful links
- If you are doing CSS for mobile you need to understand media queries, here is the W3C spec
- For CSS you might find some of these CSS guidelines useful
Browser-based developer tools
For some links on Browser-based developer tools for application debugging, optimizing, analyzing (so, we're talking about things like Firebug and Chrome devtools) see my post on Browser-based devtools
JS Web applications, Single Page Applications (SPA)
For links related to js web appdev see my primers on this topic, especially Part 2 which is loaded with useful links
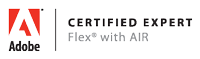
JavaScript
JavaScript appdev primers
General JavaScript
SPA Demo Application
Backbone Demo Application
Recommended sites
- Addy Osmani's blog
- Derick Bailey's Backbone posts
- Murphey's JQuery Fundamentals (original)
- Crockford's JS videos
- MSDN Project Silk
Flex/AIR
Flex/AIR primers
- Primer on Flex/AIR Multiscreen Development
- Primer on Mobile App Development w/Flex 4.5
- Primer on Flex 3 Component Lifecycle
- Primer on Flexlib MDI
Flex demo apps
all require Flash Player!
AIR mobile dev
- AIR mobile dev Tips
- AIR and Android Back key
- AIR, StageWebView, displaying local content
- AIR for Android memory issue w/large images